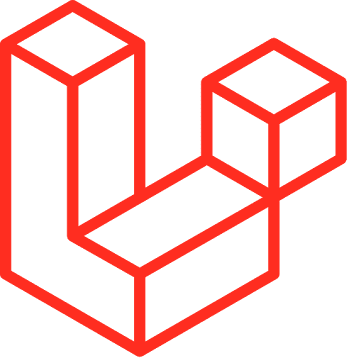
Tutorial Database Migration di Laravel:
1. Buatlah database baru lalu buka file .env di folder laravel kalian. Setelah itu sesuaikan DB_DATABASE=nama_database dengan nama database kalian.
2. Jalankan command php artisan make:migration create_contacts_table di terminal/command prompt untuk membuat file migration di dalam folder laravel kalian. Lalu akan terbentuk sebuah file baru di folder database/migrations.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateContactsTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('contacts', function (Blueprint $table) {
$table->id();
$table->string('email', 255);
$table->string('phone', 255);
$table->text('location');
$table->timestamp('created_at')->useCurrent();
$table->timestamp('updated_at')->nullable();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('contacts');
}
}
Dan ada 2 function pada file ini yaitu:
- up() yang berisi perubahan pada struktur database yang diperlukan.
- down() yang berisi operasi untuk membatalkan perubahan struktural yang dilakukan pada fungsi up().
Dan masih ada juga beberapa method yang bisa kamu gunakan untuk membuat column lain pada table migrations:
Command | Description |
$table->bigIncrements('id'); | Incrementing ID (primary key) using a "UNSIGNED BIG INTEGER" equivalent. |
$table->bigInteger('votes'); | BIGINT equivalent for the database. |
$table->binary('data'); | BLOB equivalent for the database. |
$table->boolean('confirmed'); | BOOLEAN equivalent for the database. |
$table->char('name', 4); | CHAR equivalent with a length. |
$table->date('created_at'); | DATE equivalent for the database. |
$table->dateTime('created_at'); | DATETIME equivalent for the database. |
$table->dateTimeTz('created_at'); | DATETIME (with timezone) equivalent for the database. |
$table->decimal('amount', 5, 2); | DECIMAL equivalent with a precision and scale. |
$table->double('column', 15, 8); | DOUBLE equivalent with precision, 15 digits in total and 8 after the decimal point. |
$table->enum('choices', ['foo', 'bar']); | ENUM equivalent for the database. |
$table->float('amount'); | FLOAT equivalent for the database. |
$table->increments('id'); | Incrementing ID (primary key) using a "UNSIGNED INTEGER" equivalent. |
$table->integer('votes'); | INTEGER equivalent for the database. |
$table->ipAddress('visitor'); | IP address equivalent for the database. |
$table->json('options'); | JSON equivalent for the database. |
$table->jsonb('options'); | JSONB equivalent for the database. |
$table->longText('description'); | LONGTEXT equivalent for the database. |
$table->macAddress('device'); | MAC address equivalent for the database. |
$table->mediumInteger('numbers'); | MEDIUMINT equivalent for the database. |
$table->mediumText('description'); | MEDIUMTEXT equivalent for the database. |
$table->morphs('taggable'); | Adds INTEGER taggable_id and STRING taggable_type. |
$table->nullableTimestamps(); | Same as timestamps(), except allows NULLs. |
$table->rememberToken(); | Adds remember_token as VARCHAR(100) NULL. |
$table->smallInteger('votes'); | SMALLINT equivalent for the database. |
$table->softDeletes(); | Adds deleted_at column for soft deletes. |
$table->string('email'); | VARCHAR equivalent column. |
$table->string('name', 100); | VARCHAR equivalent with a length. |
$table->text('description'); | TEXT equivalent for the database. |
$table->time('sunrise'); | TIME equivalent for the database. |
$table->timeTz('sunrise'); | TIME (with timezone) equivalent for the database. |
$table->tinyInteger('numbers'); | TINYINT equivalent for the database. |
$table->timestamp('added_on'); | TIMESTAMP equivalent for the database. |
$table->timestampTz('added_on'); | TIMESTAMP (with timezone) equivalent for the database. |
$table->timestamps(); | Adds created_at and updated_at columns. |
$table->uuid('id'); | UUID equivalent for the database. |
Credit to laravel’s documentation.
Komentar
Posting Komentar